Installation
First steps
The GSD Software Development Kit is a standalone solution which can be incorporated to any project. It can be done in a few simple steps.
At the beginning it is necessary to create a new project.
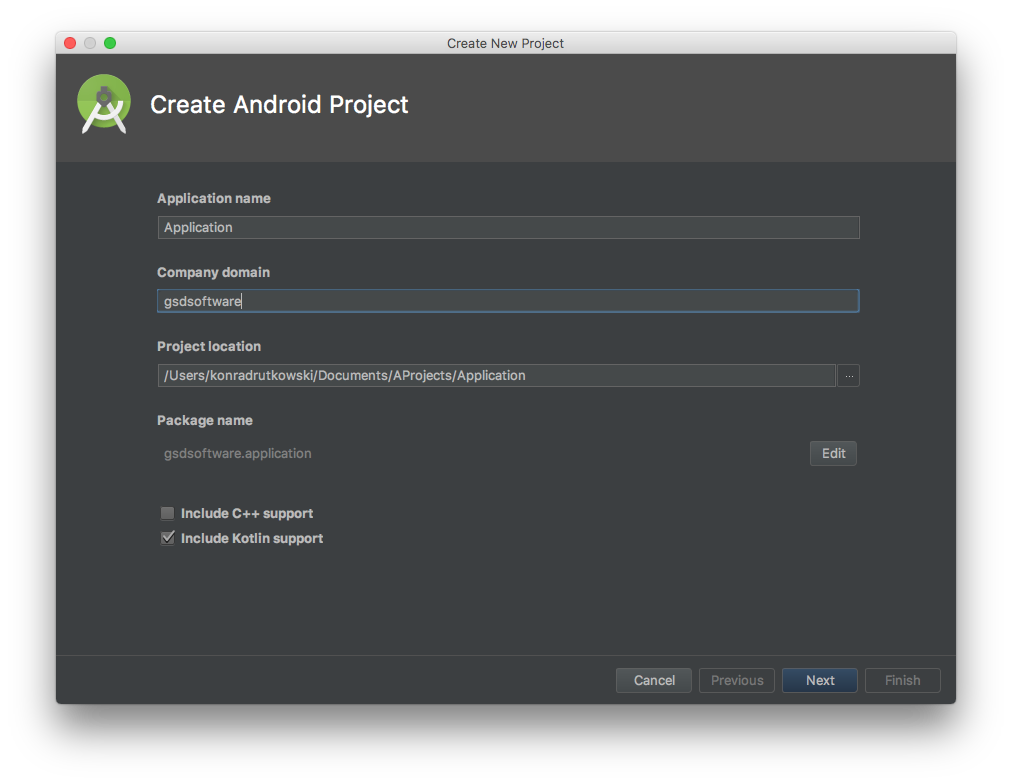
In the next steps it will be necessary to implement several dependencies and create three additional classes that are shown below in the project structure.
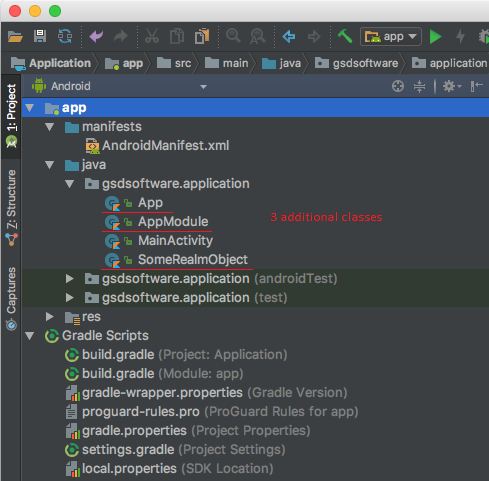
gradle dependencies
build.gradle (Project: Application)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | // Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { ext.kotlin_version = '1.1.60' repositories { google() jcenter() maven { url "http://172.16.17.30:9795/repository/maven-snapshots/" } } dependencies { classpath 'com.android.tools.build:gradle:3.0.0-beta6' classpath "io.realm:realm-gradle-plugin:3.3.1" classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { google() jcenter() maven { url "http://172.16.17.30:9795/repository/maven-snapshots/" } maven { url "https://oss.sonatype.org/content/repositories/snapshots" } } } task clean(type: Delete) { delete rootProject.buildDir } |
build.gradle (Module: app)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | apply plugin: 'com.android.application' apply plugin: 'kotlin-android' apply plugin: 'kotlin-android-extensions' apply plugin: 'realm-android' android { compileSdkVersion 26 buildToolsVersion "27.0.0" defaultConfig { applicationId "gsdsoftware.application" minSdkVersion 15 targetSdkVersion 26 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } packagingOptions { exclude 'META-INF/DEPENDENCIES' exclude 'META-INF/NOTICE' exclude 'META-INF/LICENSE' exclude 'META-INF/LICENSE.txt' exclude 'META-INF/NOTICE.txt' } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.android.support.constraint:constraint-layout:1.0.2' testImplementation 'junit:junit:4.12' androidTestImplementation('com.android.support.test.espresso:espresso-core:3.0.1', { exclude group: 'com.android.support', module: 'support-annotations' }) implementation "org.jetbrains.kotlin:kotlin-stdlib-jre7:$kotlin_version" implementation 'com.gsd.software:androidsdk:1.1.94-SNAPSHOT' } |
App Manifest
AndroidManifest.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="gsdsoftware.application"> <application android:name=".App" android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> |
Additional classes
App.kt
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | package gsdsoftware.application import android.app.Application import com.google.firebase.FirebaseApp import com.gsd.software.sdk.netconnector.GSDServicesDatabaseModule import io.realm.Realm import io.realm.RealmConfiguration class App : Application() { override fun onCreate() { super.onCreate() initRealm() initFirebase() } private fun initRealm() { Realm.init(this) val config = RealmConfiguration.Builder() .name("app.realm") .deleteRealmIfMigrationNeeded() .modules(AppModule(), GSDServicesDatabaseModule()) .build() Realm.setDefaultConfiguration(config) } private fun initFirebase() { FirebaseApp.initializeApp(this) } } |
AppModule.kt
1 2 3 4 5 6 7 | package gsdsoftware.application import io.realm.annotations.RealmModule @RealmModule(allClasses = true) class AppModule |
SomeRealmObject.kt
1 2 3 4 5 6 7 8 9 | package gsdsoftware.application import io.realm.RealmObject open class SomeRealmObject : RealmObject(){ var someRealmValue: String? = null } |
If you create another realm object, the above one can be removed. After completing all the steps contained in the documentation, you can start develop.